whatisthis?
javaScript. Array(배열) 본문
✔ Description
Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations.
Neither the length of a JavaScript array nor the types of its elements are fixed.
Since an array's length can change at any time, and data can be stored at non-contiguous locations in the array,
JavaScript arrays are not guaranteed to be dense;
this depends on how the programmer chooses to use them.
In general, these are convenient characteristics;
but if these features are not desirable for your particular use, you might consider using typed arrays.
✔ 배열의 선언 (declare)
const ArrayName = [item1, item2];
✔ 배열 item에 접근
console.log(ArrayName[0]);
인덱스 number로 배열 아이템에 접근할 수 있다.
+) 응용
const lastItem = ArrayName[ArrayName.length - 1];
// index는 0번지부터 시작이므로, 마지막 아이템의 index는 length-1이다.
.length는 메서드가 아닌 프로퍼티(속성)으로, 반환값은 부호 없는 32비트 정수이다.
✔ 배열 item마다 실행 - ForEach()함수
방법1. 외부 함수 이용
arrayName.forEach(functionName);
function functionName(item) {
console.log("This item is " + item);
addEventListener에서 event라는 프로퍼티를 기본 제공받듯이,
forEach에서는 어떤 아이템이 실행된 것인지 확인할 수 있는 item 프로퍼티가 있음.
(item / index / array 가 있음)
방법2. 직접 괄호안에 선언
arrayName.forEach(function (item) {
console.log("This item is " + item);
})
// Arrow Function 이용
arrayName.forEach((item) => console.log("This item is ", item));
✔ 배열 item 추가
- 배열 끝에 항목 추가
arrayName.push('item');
- 배열 앞에 항목 추가
arrayName.unshift('item');
✔ 배열 item 제거
- 배열 끝에서부터 제거
arrayName.pop();
- 배열 앞에서부터 제거
arrayName.shift();
지금까지 내용을 활용한 예제 🔻
const arrayName = ['item1', 'item2'];
console.log(arrayName[0]);
console.log(arrayName[arrayName.length - 1]);
arrayName.forEach((item) => console.log("This item is " + item));
arrayName.push('back');
arrayName.unshift('front');
console.log(arrayName);
arrayName.pop();
console.log(arrayName);
arrayName.shift();
console.log(arrayName);
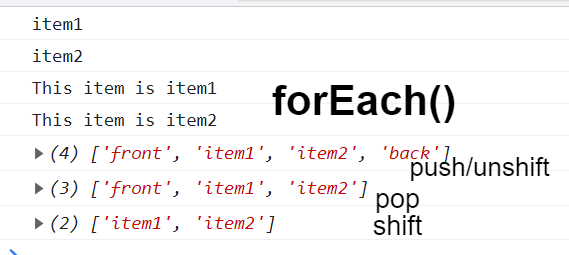
REFERENCE
https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array
Array - JavaScript | MDN
JavaScript Array 전역 객체는 배열을 생성할 때 사용하는 리스트 형태의 고수준 객체입니다.
developer.mozilla.org
'WEB STUDY > JAVASCRIPT' 카테고리의 다른 글
javaScript. break문 (0) | 2021.12.20 |
---|---|
javaScript(Node.js). readline과 fs모듈 (0) | 2021.12.06 |
javaScript. Math.floor과 Math.trunc의 차이 (0) | 2021.12.06 |
javaScript. 호이스팅(Hoisting) 이란? (0) | 2021.12.06 |
Momentum clone (part final) (0) | 2021.11.28 |